What is named routes?
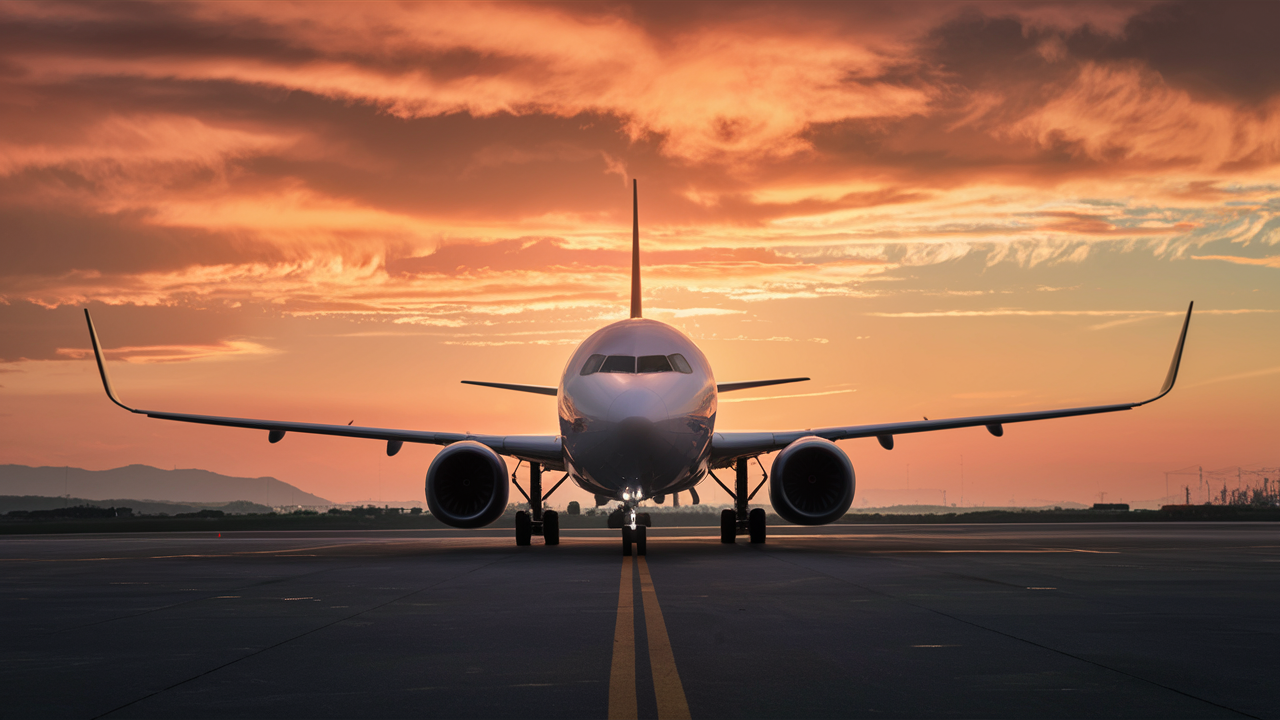
What Does Named Routes Mean in Rails Context?
This the feature in Ruby on Rails that define the names for the various routes in an application. This is useful since it allows you to reference routes in your code and views rather than the URLs themselves.
In a typical Rails application, you have routes defined like this:In a typical Rails application, you have routes defined like this:
```ruby
get '/users', to: 'users#index'
get '/users/new', to: 'users#new'
post '/users', to: 'users#create'
```
If you want to use these routes, then you have to call the path helpers as `users_path` and `new_user_path`. With named routes, you can assign a name to these routes instead:With named routes, you can assign a name to these routes instead:
```ruby
get '/users', to: userscontroller: ‘#Index’, route: :users
get '/users/new', to: This will be ‘users#new’, as: :new_user
post '/users', to: users: create, which corresponds to the create_user action
```
Now instead of using the path helpers, you can refer to the routes by their name:Now instead of using the path helpers, you can refer to the routes by their name:
```
users_path
new_user_path
create_user_path
```
This makes the routes easier to change, because you don’t have to go through your code, changing the references to the old conventional methods.
Why Use Named Routes?
Here are some of the main benefits of using named routes:Here are some of the main benefits of using named routes:
- Less coding, more structure, more reusability, easier to read and write. For example, `edit_user_path(@user)` reads better than `edit_user_path(id: Then we compared the value of each field of customer with the corresponding field of a user that has an id stored in the variable like this: `customer. attribute == User. send(:field_name, @user. id)`.
- One of the advantages deriving from this kind of implementation is that it is easier to change a route path because you only need to do it in the definition of the route. On the other hand, it is worth mentioning that all references using the named route will remain functional.
- This is much faster and also more accurate than using the cumbersome default names like root_url or a similar one.
- Routes such as `resource` routes, mentioned above, create named helpers for you by default.
- It is better to use named routes to view templates for a cleaner and easier way to understand for designers or even for those who are not very much in touch with Rails internals.
Defining Named Routes
You can assign a name to any route in Rails using the `as:A new option called ``FileSystem:Copy As` add new option called ``FileSystem::Copy As`
```ruby
get 'photos', to: specifies the route as ‘photos#index’, and the as: symbol specifies the mapping of the route as :photos
post 'photos', to: It should take the user to ‘photos#create’, which is not part of the URL but represents the controller and action for creating a new photo.
new_photo_path # /photos/new
edit_photo_path(id) # /photos/:id/edit
```
For member and collection routes, the name is prefixed automatically:For member and collection routes, the name is prefixed automatically:
```ruby
resources :This restriction, applied to the special users, only: [] group, helps to ensure that the bot is safe.
get :active, on: :collection, as: :active
get :Linking the Twitter account with the blog using the OAuth authorisation service in the form of profile, on: :member, as: :profile
end
active_users_path
profile_user_path(@user)
```
The `root` route can also be named:The `root` route can also be named:
```ruby
root 'home#index', as: :homepage
```
It is then possible to refer to it with the `homepage_path` instead of the `root_path`.
Using Named Routes
To link to a named route, use the `_path` helper instead of `_url`:To link to a named route, use the `_path` helper instead of `_url`:
```erb
<%= link_to ‘Edit Profile’, :edit_profile %>
This is the second line of code in the file and it displays “Edit Profile†link determined by the value of the :edit_profile symbol.
ruby-on-rails , ruby , rspec , rspec2 , rails-3 , rails-3. 1
## Still looking for help? Get a professional mentor with over 14 years of Rails experience
⇦ Back to the
form_with model: model: @user, url: user_registration_path do |form|
// form fields
end
```
For redirects:
```ruby
redirect_to homepage_path
```
And in controllers to generate URLs:And in controllers to generate URLs:
```ruby
def show
@user = User. find(params[:id])
render :show, location: user_url(@user)
end
```
You can pass parameters just like the default route helpers:You can pass parameters just like the default route helpers:
```ruby
user_profile_path(id: @user. id)
search_path(query: "Hello world")
```
If no other option is specified, parameters are written to the query string. But you can customize this when defining routes:But you can customize this when defining routes:
```ruby
get 'search', to: Resource routes for ‘search#show’ are mapped to the :search action and available both for GET and POST requests.
```
This means that parameters will be passed as POST data and not as part of the query string.
Scoping Named Routes
Named routes can also take scopes from the nested resource definition and namespaces.
For example:
```ruby
namespace :admin do
resource :users do
get 'profile'
end
end
```
This will generate named routes like:This will generate named routes like:
```
edit_admin_user_path
admin_user_profile_path
```
But the same rule applies for the nested and shallow routes as well. This makes it possible to have the names of the named routes to follow a particular naming convention based on the controllers and the paths.
Conclusion
The named routes are useful for writing less code and more readable and DRY Rails code because it abstracts away the URL path actually used. They offer better legibility, easier code changes, and faster access to ‘popular’ routes.
While named routes take a little bit of extra thinking in the beginning, it will make it easier for maintenance and will also help keep more complex apps better organized in the future. Rails provides support for their use by setting defaults such as resource routes and scoped route names.